Custom commands make Slack more awesome
By:
Mark Biek
on 12/7/2016
Slack is already awesome, but you can make it even better with a custom Slash Command.
What are Slash Commands?
Slash Commands are special commands you type into Slack to do lots of handy things.
There are some built-in commands like:
/away
to toggle your Away status/invite @user
to invite a user to a channel/leave
to leave a channel
(There are lots of others. Type “/"
into Slack to see a complete list).
Creating a custom command
It’s easy to write your own commands to do just about anything. We have a few here at VIA, for example /whereis @user
to show if a person is in a meeting, or /tick
to check for incomplete Tick time sheets.
To create a new command, start by launching Apps & integrations from inside Slack.
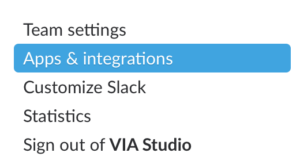
Make sure you have the correct Slack team selected and click on Manage.
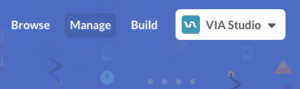
Then click Custom Integrations and the Slash Commands.
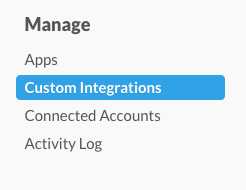
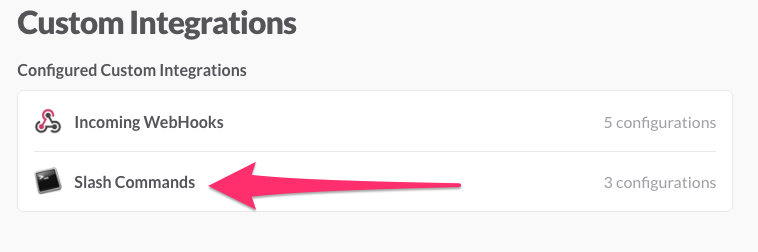
Lastly, click Add Configuration to create your new command.
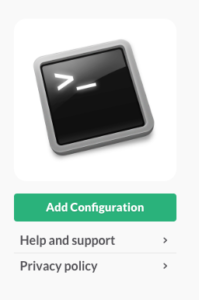
You’ll need to fill in a few settings for your command, first is the name of your command; this is what your users will type into Slack.
You also need to specify the URL that is called and the request method (GET or POST). The url being called can be hosted anywhere and written in any language. We’ll be using PHP for our example.
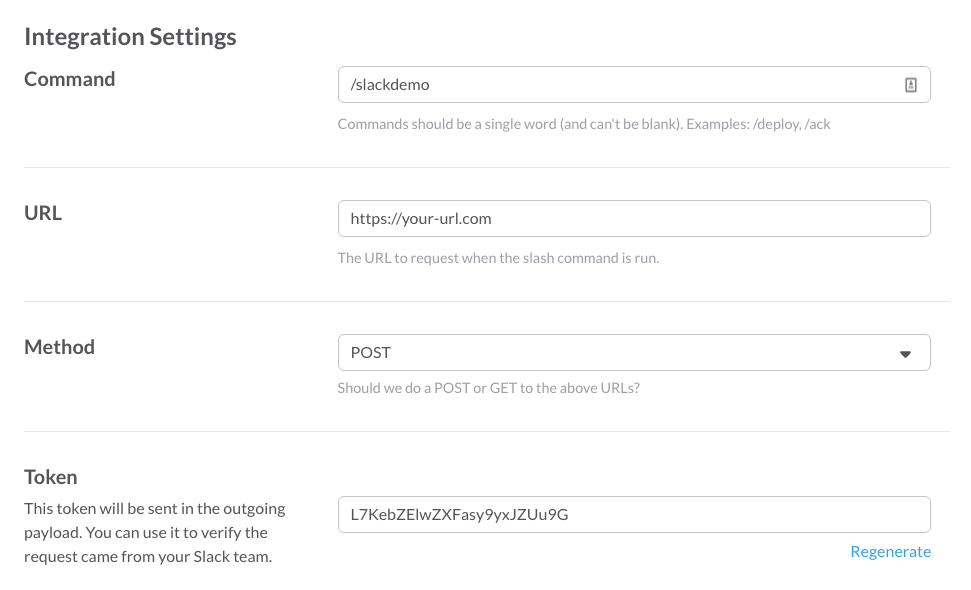
You also get a randomly generated token used to validate requests to your URL (to make sure they’re actually coming from Slack), plus some optional settings like a custom icon or description.
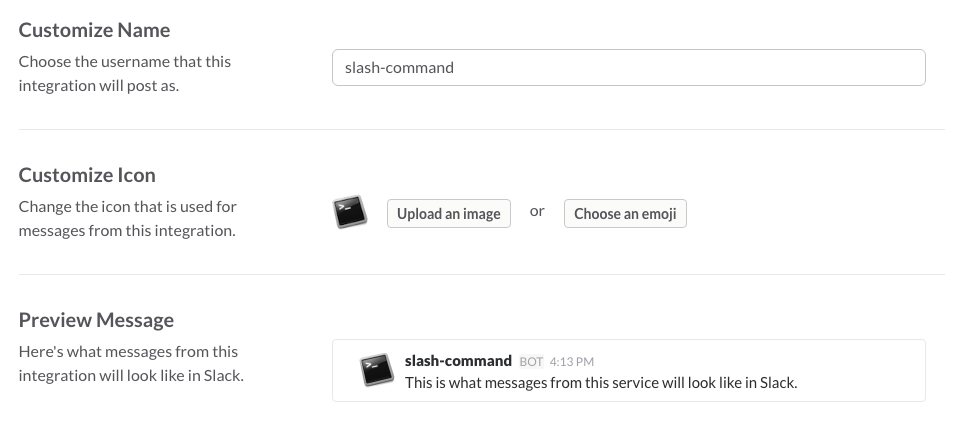
Let’s look at some code
When a command is executed, the following data is sent to the command url:
- token (The token from the command configuration)
- team_id (The id of the Slack team)
- channel_id (The id of the channel where the command was executed)
- channel_name (The name of the channel where the command was executed)
- user_id (The id of the user who ran the command)
- user_name (The name of the user who ran the command)
- command (The command that was executed)
- text (Any text typed after the command-name)
- response_url (A url that can receive additional responses)
Here’s a “Hello World” example which validates the token and prints out Hello World!
A slightly more advanced example
Chances are, your command is going do more than just print out a simple message. You might need to query a database or access an external API which can take a little bit of time.
Slack will only wait for 3000 milliseconds for your URL to respond before throwing a Timeout Error. That means you’ll want to give an initial response (to tell the user something is happening) and then respond again when the command’s work is finished
Our next command will immediately respond to the user, then continue processing.
This little block of code is the key:ignore_user_abort(true);
ob_start();
echo('{ "text": ":timer_clock: Doing some stuff, please wait..."}');
header($_SERVER["SERVER_PROTOCOL"] . " 200 OK");
header("Content-Type: application/json");
header('Content-Length: ' . ob_get_length());
ob_end_flush();
ob_flush();
flush();
This outputs a message to the user (and returns an HTTP/200 status code for success). The output is immediately sent but the script will keep executing, even after the connection has ended.
Once we’re ready to send the final result back to the user, we use cURL to send a JSON-encoded response to the response_url.
Fancy output
The above output is pretty basic. A simple line of text isn’t very interesting and doesn’t work well for a longer response.
First, you can add any Slack emoji to the response by including it in the text (for example, 'Hello World! :thumbsup:'
).
You can also do lists of items, even having items in different colors!
$payload = [
'text' => 'All done! :thumbsup:',
'attachments' => [
['text' => 'Thing 1', 'color' => 'success'],
['text' => 'Thing 2', 'color' => 'danger'],
['text' => 'Thing 3', 'color' => 'warning'],
['text' => 'Thing 4', 'color' => '#0000ff']
]
];
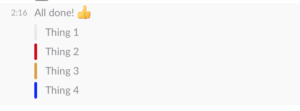
Go forth and write nifty commands for your team
There’s lots of good documentation on custom commands. Go out there and write something to make life easier for your team!
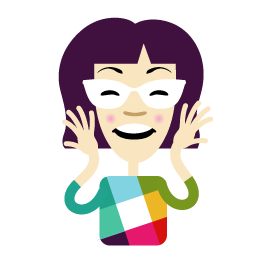
Related Posts
Developing the New via.studio
By:Alec Robertson on 6/14/2021
A deep dive into creating a fast, user-focused experience on the latest web technology stack for the new via.studio website.
Read More »Intro To Figma Dev Mode
By:Morgan Plappert on 7/1/2024
I’ve always loved Figma for the fact that every update is driven by a desire to ease handoff from design to development. But what if there was no handoff? What if developers become a part of the design process? Is Figma’s new Developer Mode the answer to this? I’m hopeful it’s a step in the right direction…
Read More »